|
|
Teach Yourself SQL in 21 Days, Second Edition
 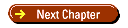
- Appendix B -
Source Code Listings for the C++ Program Used on Day 14
// tyssqvw.h : interface of the CTyssqlView class
//
/////////////////////////////////////////////////////////////////////////////
class CTyssqlSet;
class CTyssqlView : public CRecordView
{
protected: // create from serialization only
CTyssqlView();
DECLARE_DYNCREATE(CTyssqlView)
public:
//{{AFX_DATA(CTyssqlView)
enum { IDD = IDD_TYSSQL_FORM };
CTyssqlSet* m_pSet;
//}}AFX_DATA
// Attributes
public:
CTyssqlDoc* GetDocument();
// Operations
public:
virtual CRecordset* OnGetRecordset();
// Implementation
public:
virtual ~CTyssqlView();
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
protected:
virtual void DoDataExchange(CDataExchange* pDX);// DDX/DDV support
virtual void OnInitialUpdate(); // called first time after construct
// Generated message map functions
protected:
//{{AFX_MSG(CTyssqlView)
// NOTE - the ClassWizard will add and remove member functions here.
// DO NOT EDIT what you see in these blocks of generated code !
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
#ifndef _DEBUG // debug version in tyssqvw.cpp
inline CTyssqlDoc* CTyssqlView::GetDocument()
{ return (CTyssqlDoc*)m_pDocument; }
#endif
/////////////////////////////////////////////////////////////////////////////
// tyssql.h : main header file for the TYSSQL application
//
#ifndef __AFXWIN_H__
#error include 'stdafx.h' before including this file for PCH
#endif
#include "resource.h" // main symbols
/////////////////////////////////////////////////////////////////////////////
// CTyssqlApp:
// See tyssql.cpp for the implementation of this class
//
class CTyssqlApp : public CWinApp
{
public:
CTyssqlApp();
// Overrides
virtual BOOL InitInstance();
// Implementation
//{{AFX_MSG(CTyssqlApp)
afx_msg void OnAppAbout();
// NOTE - the ClassWizard will add and remove member functions here.
// DO NOT EDIT what you see in these blocks of generated code !
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
/////////////////////////////////////////////////////////////////////////////
// tyssqset.h : interface of the CTyssqlSet class
//
/////////////////////////////////////////////////////////////////////////////
class CTyssqlSet : public CRecordset
{
DECLARE_DYNAMIC(CTyssqlSet)
public:
CTyssqlSet(CDatabase* pDatabase = NULL);
// Field/Param Data
//{{AFX_FIELD(CTyssqlSet, CRecordset)
CString m_NAME;
CString m_ADDRESS;
CString m_STATE;
CString m_ZIP;
CString m_PHONE;
CString m_REMARKS;
//}}AFX_FIELD
// Implementation
protected:
virtual CString GetDefaultConnect(); // Default connection string
virtual CString GetDefaultSQL(); // default SQL for Recordset
virtual void DoFieldExchange(CFieldExchange* pFX); // RFX support
};
// tyssqdoc.h : interface of the CTyssqlDoc class
//
/////////////////////////////////////////////////////////////////////////////
class CTyssqlDoc : public CDocument
{
protected: // create from serialization only
CTyssqlDoc();
DECLARE_DYNCREATE(CTyssqlDoc)
// Attributes
public:
CTyssqlSet m_tyssqlSet;
// Operations
public:
// Implementation
public:
virtual ~CTyssqlDoc();
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
protected:
virtual BOOL OnNewDocument();
// Generated message map functions
protected:
//{{AFX_MSG(CTyssqlDoc)
// NOTE - the ClassWizard will add and remove member functions here.
// DO NOT EDIT what you see in these blocks of generated code !
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
/////////////////////////////////////////////////////////////////////////////
// stdafx.h : include file for standard system include files,
// or project specific include files that are used frequently, but
// are changed infrequently
//
#include <afxwin.h> // MFC core and standard components
#include <afxext.h> // MFC extensions (including VB)
#include <afxdb.h> // MFC database classes
////////////////////////////////////////////////////////////
//{{NO_DEPENDENCIES}}
// App Studio generated include file.
// Used by TYSSQL.RC
//
#define IDR_MAINFRAME 2
#define IDD_ABOUTBOX 100
#define IDD_TYSSQL_FORM 101
#define IDP_FAILED_OPEN_DATABASE 103
#define IDC_NAME 1000
#define IDC_ADDRESS 1001
#define IDC_STATE 1002
#define IDC_ZIP 1003
// Next default values for new objects
//
#ifdef APSTUDIO_INVOKED
#ifndef APSTUDIO_READONLY_SYMBOLS
#define _APS_NEXT_RESOURCE_VALUE 102
#define _APS_NEXT_COMMAND_VALUE 32771
#define _APS_NEXT_CONTROL_VALUE 1004
#define _APS_NEXT_SYMED_VALUE 101
#endif
#endif
///////////////////////////////////////////////////
// mainfrm.h : interface of the CMainFrame class
//
/////////////////////////////////////////////////////////////////////////////
class CMainFrame : public CFrameWnd
{
protected: // create from serialization only
CMainFrame();
DECLARE_DYNCREATE(CMainFrame)
// Attributes
public:
// Operations
public:
// Implementation
public:
virtual ~CMainFrame();
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
protected: // control bar embedded members
CStatusBar m_wndStatusBar;
CToolBar m_wndToolBar;
// Generated message map functions
protected:
//{{AFX_MSG(CMainFrame)
afx_msg int OnCreate(LPCREATESTRUCT lpCreateStruct);
// NOTE - the ClassWizard will add and remove member functions here.
// DO NOT EDIT what you see in these blocks of generated code!
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
/////////////////////////////////////////////////////////////////////////////
// tyssqvw.cpp : implementation of the CTyssqlView class
//
#include "stdafx.h"
#include "tyssql.h"
#include "tyssqset.h"
#include "tyssqdoc.h"
#include "tyssqvw.h"
#ifdef _DEBUG
#undef THIS_FILE
static char BASED_CODE THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CTyssqlView
IMPLEMENT_DYNCREATE(CTyssqlView, CRecordView)
BEGIN_MESSAGE_MAP(CTyssqlView, CRecordView)
//{{AFX_MSG_MAP(CTyssqlView)
// NOTE - the ClassWizard will add and remove mapping macros here.
// DO NOT EDIT what you see in these blocks of generated code!
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTyssqlView construction/destruction
CTyssqlView::CTyssqlView()
: CRecordView(CTyssqlView::IDD)
{
//{{AFX_DATA_INIT(CTyssqlView)
m_pSet = NULL;
//}}AFX_DATA_INIT
// TODO: add construction code here
}
CTyssqlView::~CTyssqlView()
{
}
void CTyssqlView::DoDataExchange(CDataExchange* pDX)
{
CRecordView::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CTyssqlView)
DDX_FieldText(pDX, IDC_ADDRESS, m_pSet->m_ADDRESS, m_pSet);
DDX_FieldText(pDX, IDC_NAME, m_pSet->m_NAME, m_pSet);
DDX_FieldText(pDX, IDC_STATE, m_pSet->m_STATE, m_pSet);
DDX_FieldText(pDX, IDC_ZIP, m_pSet->m_ZIP, m_pSet);
//}}AFX_DATA_MAP
}
void CTyssqlView::OnInitialUpdate()
{
m_pSet = &GetDocument()->m_tyssqlSet;
CRecordView::OnInitialUpdate();
}
/////////////////////////////////////////////////////////////////////////////
// CTyssqlView diagnostics
#ifdef _DEBUG
void CTyssqlView::AssertValid() const
{
CRecordView::AssertValid();
}
void CTyssqlView::Dump(CDumpContext& dc) const
{
CRecordView::Dump(dc);
}
CTyssqlDoc* CTyssqlView::GetDocument() // non-debug version is inline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CTyssqlDoc)));
return (CTyssqlDoc*)m_pDocument;
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CTyssqlView database support
CRecordset* CTyssqlView::OnGetRecordset()
{
return m_pSet;
}
/////////////////////////////////////////////////////////////////////////////
// CTyssqlView message handlers
// tyssqset.cpp : implementation of the CTyssqlSet class
//
#include "stdafx.h"
#include "tyssql.h"
#include "tyssqset.h"
/////////////////////////////////////////////////////////////////////////////
// CTyssqlSet implementation
IMPLEMENT_DYNAMIC(CTyssqlSet, CRecordset)
CTyssqlSet::CTyssqlSet(CDatabase* pdb)
: CRecordset(pdb)
{
//{{AFX_FIELD_INIT(CTyssqlSet)
m_NAME = "";
m_ADDRESS = "";
m_STATE = "";
m_ZIP = "";
m_PHONE = "";
m_REMARKS = "";
m_nFields = 6;
//}}AFX_FIELD_INIT
}
CString CTyssqlSet::GetDefaultConnect()
{
return "ODBC;DSN=TYSSQL;";
}
CString CTyssqlSet::GetDefaultSQL()
{
return "SELECT * FROM CUSTOMER ORDER BY NAME";
}
void CTyssqlSet::DoFieldExchange(CFieldExchange* pFX)
{
//{{AFX_FIELD_MAP(CTyssqlSet)
pFX->SetFieldType(CFieldExchange::outputColumn);
RFX_Text(pFX, "NAME", m_NAME);
RFX_Text(pFX, "ADDRESS", m_ADDRESS);
RFX_Text(pFX, "STATE", m_STATE);
RFX_Text(pFX, "ZIP", m_ZIP);
RFX_Text(pFX, "PHONE", m_PHONE);
RFX_Text(pFX, "REMARKS", m_REMARKS);
//}}AFX_FIELD_MAP
}
// tyssql.cpp : Defines the class behaviors for the application.
//
#include "stdafx.h"
#include "tyssql.h"
#include "mainfrm.h"
#include "tyssqset.h"
#include "tyssqdoc.h"
#include "tyssqvw.h"
#ifdef _DEBUG
#undef THIS_FILE
static char BASED_CODE THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CTyssqlApp
BEGIN_MESSAGE_MAP(CTyssqlApp, CWinApp)
//{{AFX_MSG_MAP(CTyssqlApp)
ON_COMMAND(ID_APP_ABOUT, OnAppAbout)
// NOTE - the ClassWizard will add and remove mapping macros here.
// DO NOT EDIT what you see in these blocks of generated code!
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTyssqlApp construction
CTyssqlApp::CTyssqlApp()
{
// TODO: add construction code here,
// Place all significant initialization in InitInstance
}
/////////////////////////////////////////////////////////////////////////////
// The one and only CTyssqlApp object
CTyssqlApp NEAR theApp;
/////////////////////////////////////////////////////////////////////////////
// CTyssqlApp initialization
BOOL CTyssqlApp::InitInstance()
{
// Standard initialization
// If you are not using these features and wish to reduce the size
// of your final executable, you should remove from the following
// the specific initialization routines you do not need.
SetDialogBkColor(); // Set dialog background color to gray
LoadStdProfileSettings(); // Load standard INI file options (including MRU)
// Register the application's document templates. Document templates
// serve as the connection between documents, frame windows and views.
CSingleDocTemplate* pDocTemplate;
pDocTemplate = new CSingleDocTemplate(
IDR_MAINFRAME,
RUNTIME_CLASS(CTyssqlDoc),
RUNTIME_CLASS(CMainFrame), // main SDI frame window
RUNTIME_CLASS(CTyssqlView));
AddDocTemplate(pDocTemplate);
// create a new (empty) document
OnFileNew();
if (m_lpCmdLine[0] != '\0')
{
// TODO: add command line processing here
}
return TRUE;
}
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
//{{AFX_DATA(CAboutDlg)
enum { IDD = IDD_ABOUTBOX };
//}}AFX_DATA
// Implementation
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//{{AFX_MSG(CAboutDlg)
// No message handlers
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CAboutDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
//{{AFX_MSG_MAP(CAboutDlg)
// No message handlers
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
// App command to run the dialog
void CTyssqlApp::OnAppAbout()
{
CAboutDlg aboutDlg;
aboutDlg.DoModal();
}
/////////////////////////////////////////////////////////////////////////////
// CTyssqlApp commands
// tyssqdoc.cpp : implementation of the CTyssqlDoc class
//
#include "stdafx.h"
#include "tyssql.h"
#include "tyssqset.h"
#include "tyssqdoc.h"
#ifdef _DEBUG
#undef THIS_FILE
static char BASED_CODE THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CTyssqlDoc
IMPLEMENT_DYNCREATE(CTyssqlDoc, CDocument)
BEGIN_MESSAGE_MAP(CTyssqlDoc, CDocument)
//{{AFX_MSG_MAP(CTyssqlDoc)
// NOTE - the ClassWizard will add and remove mapping macros here.
// DO NOT EDIT what you see in these blocks of generated code!
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTyssqlDoc construction/destruction
CTyssqlDoc::CTyssqlDoc()
{
// TODO: add one-time construction code here
}
CTyssqlDoc::~CTyssqlDoc()
{
}
BOOL CTyssqlDoc::OnNewDocument()
{
if (!CDocument::OnNewDocument())
return FALSE;
// TODO: add reinitialization code here
// (SDI documents will reuse this document)
return TRUE;
}
/////////////////////////////////////////////////////////////////////////////
// CTyssqlDoc diagnostics
#ifdef _DEBUG
void CTyssqlDoc::AssertValid() const
{
CDocument::AssertValid();
}
void CTyssqlDoc::Dump(CDumpContext& dc) const
{
CDocument::Dump(dc);
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CTyssqlDoc commands
// stdafx.cpp : source file that includes just the standard includes
// stdafx.pch will be the pre-compiled header
// stdafx.obj will contain the pre-compiled type information
#include "stdafx.h"
// mainfrm.cpp : implementation of the CMainFrame class
//
#include "stdafx.h"
#include "tyssql.h"
#include "mainfrm.h"
#ifdef _DEBUG
#undef THIS_FILE
static char BASED_CODE THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CMainFrame
IMPLEMENT_DYNCREATE(CMainFrame, CFrameWnd)
BEGIN_MESSAGE_MAP(CMainFrame, CFrameWnd)
//{{AFX_MSG_MAP(CMainFrame)
// NOTE - the ClassWizard will add and remove mapping macros here.
// DO NOT EDIT what you see in these blocks of generated code !
ON_WM_CREATE()
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// arrays of IDs used to initialize control bars
// toolbar buttons - IDs are command buttons
static UINT BASED_CODE buttons[] =
{
// same order as in the bitmap 'toolbar.bmp'
ID_EDIT_CUT,
ID_EDIT_COPY,
ID_EDIT_PASTE,
ID_SEPARATOR,
ID_FILE_PRINT,
ID_SEPARATOR,
ID_RECORD_FIRST,
ID_RECORD_PREV,
ID_RECORD_NEXT,
ID_RECORD_LAST,
ID_SEPARATOR,
ID_APP_ABOUT,
};
static UINT BASED_CODE indicators[] =
{
ID_SEPARATOR, // status line indicator
ID_INDICATOR_CAPS,
ID_INDICATOR_NUM,
ID_INDICATOR_SCRL,
};
/////////////////////////////////////////////////////////////////////////////
// CMainFrame construction/destruction
CMainFrame::CMainFrame()
{
// TODO: add member initialization code here
}
CMainFrame::~CMainFrame()
{
}
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
if (!m_wndToolBar.Create(this) ||
!m_wndToolBar.LoadBitmap(IDR_MAINFRAME) ||
!m_wndToolBar.SetButtons(buttons,
sizeof(buttons)/sizeof(UINT)))
{
TRACE("Failed to create toolbar\n");
return -1; // fail to create
}
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators)/sizeof(UINT)))
{
TRACE("Failed to create status bar\n");
return -1; // fail to create
}
return 0;
}
/////////////////////////////////////////////////////////////////////////////
// CMainFrame diagnostics
#ifdef _DEBUG
void CMainFrame::AssertValid() const
{
CFrameWnd::AssertValid();
}
void CMainFrame::Dump(CDumpContext& dc) const
{
CFrameWnd::Dump(dc);
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CMainFrame message handlers
 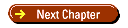
© Copyright, Macmillan Computer Publishing. All
rights reserved.
|